Data Science with Python: Getting Started with Pandas and NumPy
Welcome to the exciting world of data science with Python! Today, machine learning and artificial intelligence are changing how businesses decide. Python is the top language for data analysis, thanks to its strong libraries that make complex data easier.
Pandas is a key player in data science, giving users powerful tools for handling data. It’s an open-source library that makes cleaning, transforming, and analyzing data simple. It works well with tables, time series, and complex data sets.
NumPy adds to Pandas by offering advanced math capabilities. It has high-performance array objects and tools for math operations. Data scientists use NumPy for its fast array processing and strong computational power.
As you start your data science journey, you’ll see how Pandas and NumPy work together. They help unlock insights from raw data. Python’s ecosystem lets you turn data into useful information for making decisions.
In the next sections, we’ll explore how to use Pandas and NumPy in real-world scenarios. You’ll learn new skills and open up new career paths in data science.
Table of Contents
Understanding the Foundations of Data Analysis in Python
Python is a top choice for data science. It’s great for predictive analytics and handling big data. Its wide range of tools makes it perfect for data mining and analysis.
Data analysis follows six key steps:
- Ask or Specify Data Requirements
- Prepare or Collect Data
- Clean and Process
- Analyze
- Share
- Act or Report
Why Python Stands Out in Data Analysis
Python is known for its simplicity and strong libraries. NumPy is a key library for scientific computing. It offers high-performance arrays for complex math operations.
NumPy’s main features are:
- Multidimensional array support
- Element-wise arithmetic operations
- Advanced mathematical functions
- Efficient data processing capabilities
Core Libraries for Data Science
The Python data science world relies on strong libraries. Pandas is built on NumPy and offers advanced data tools. It has Series for one-dimensional data and DataFrame for two-dimensional data.
Getting Your Environment Ready
Setting up your Python environment is easy. Just install libraries like NumPy, Pandas, and Matplotlib with pip or conda. These tools will help you start data science projects.
Getting Started with Pandas Library
Pandas is a strong Python library that changes how we work with data. It’s built on NumPy and offers tools for complex datasets. This makes data handling more efficient.
Starting your data science journey means learning two main Pandas tools:
- Series: A one-dimensional labeled array that can hold different data types
- DataFrame: A two-dimensional labeled data structure like a spreadsheet
Pandas makes data prep easy. You can read data from CSV, Excel, and SQL databases. It has methods for exploring and cleaning data, which is key for advanced analytics and machine learning.
Some key Pandas commands help you get to know your data:
- `df.head()`: Shows the first 5 rows
- `df.describe()`: Gives statistical summaries
- `df.isnull().sum()`: Finds missing values
For natural language processing, Pandas is great at transforming data. You can merge datasets, deal with missing values, and get text ready for neural networks with a few lines of code.
Pandas makes complex data tasks simple, making it a must-have for data scientists and researchers.
Essential NumPy Features for Beginners
NumPy is a key library for data science and statistical modeling. It offers tools for fast numerical computing in Python. Knowing its core features can boost your skills in deep learning and data analysis.
NumPy makes working with numerical data fast and efficient. Its N-dimensional arrays (ndarray) are great for complex math operations. They speed up and improve the accuracy of your work.
Array Operations and Mathematics
NumPy has several array creation functions for easier math:
- Create zero-filled arrays using np.zeros()
- Generate arrays of ones with np.ones()
- Fill arrays with specific values using np.full()
- Create identity matrices with np.eye()
Broadcasting and Vectorization
NumPy’s broadcasting and vectorization are its strongest points. They let you work on whole arrays at once, without loops. This makes your code shorter and faster.
Operation | Description | Performance Impact |
---|---|---|
Broadcasting | Allows operations between arrays of different shapes | High computational efficiency |
Vectorization | Applies operations to entire arrays simultaneously | Significant speed improvements |
Random Number Generation
For statistical modeling and deep learning, NumPy’s random number tools are essential. The numpy.random module has many distributions and methods. They’re vital for simulations and machine learning.
Learning these NumPy features opens up powerful tools for data science and advanced programming.
Data Science Fundamentals and Workflow
Data science turns raw data into useful insights. It starts with a key workflow that helps in analyzing data across many fields. This process needs a mix of technical skills, knowledge of the field, and thinking critically.
The heart of data science is a workflow that helps organizations make smart decisions. This workflow includes several important steps:
- Problem definition and context analysis
- Data collection and acquisition
- Data cleaning and preprocessing
- Exploratory data analysis
- Feature engineering
- Model development
- Model evaluation and deployment
Machine learning and artificial intelligence are key in improving data science. They help data scientists find complex patterns and create predictive models. These models lead to new ideas in many areas.
Workflow Stage | Key Activities | Primary Tools |
---|---|---|
Data Collection | Gathering raw data from various sources | APIs, Web Scraping, Databases |
Data Cleaning | Removing inconsistencies and preparing data | Pandas, NumPy |
Analysis | Extracting meaningful insights | Statistical Libraries, Machine Learning Algorithms |
Data science experts need to know programming languages like Python, statistical methods, and specific field knowledge. They must keep learning and adapting as the field changes fast.
Data Manipulation Techniques with Pandas
Pandas is a key tool for data scientists and analysts. It helps turn raw data into useful insights. This is done through advanced data manipulation strategies.
Effective data preprocessing is vital in predictive analytics and data mining. Pandas has several methods to clean, transform, and prepare datasets. This makes them ready for deeper analysis.
Reading and Writing Data
Pandas makes importing and exporting data easy. You can work with many file formats:
- Reading CSV files using pd.read_csv()
- Importing Excel spreadsheets with pd.read_excel()
- Exporting transformed data to different formats
Filtering and Sorting Data
Data exploration is easy with Pandas’ filtering tools. You can:
- Select specific columns using .loc and .iloc
- Sort data based on multiple criteria
- Create complex queries with boolean indexing
Handling Missing Values
Missing data can affect analysis. Pandas has strong methods to handle this:
- Remove missing values with dropna()
- Fill missing values using fillna()
- Replace missing data with statistical measures like mean or median
Mastering these techniques transforms raw data into actionable insights.
Statistical Analysis Using NumPy
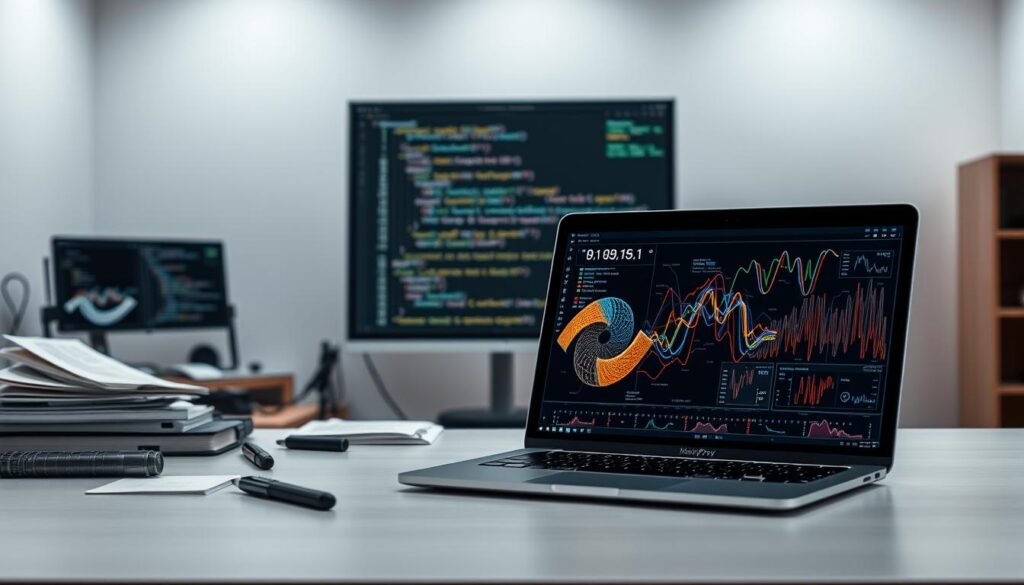
NumPy is a key tool for data scientists and researchers. It’s essential for working with neural networks and natural language processing. With NumPy, you can do complex statistical work quickly and accurately.
It has a wide range of statistical functions. These help you find important insights in your data. Here are some key measures you can calculate:
- Mean: Calculate the average value of a dataset
- Median: Find the middle value in a distribution
- Standard Deviation: Measure data variability
- Variance: Understand data spread
Statistical analysis is vital when working with neural networks and natural language processing. NumPy makes these complex tasks easier. It uses simple functions that work well with big datasets.
NumPy also offers advanced statistical techniques. These include:
- Correlation analysis
- Probability distribution generation
- Hypothesis testing
- Sampling methodologies
Data scientists can use NumPy to create detailed analytical models. It’s great for both research and machine learning. These tools help you understand your data deeply.
Data Visualization with Matplotlib and Seaborn
Data visualization makes complex data easy to understand. Python’s Matplotlib and Seaborn libraries help create beautiful graphics. They turn data insights into clear, compelling visuals.
Matplotlib and Seaborn offer tools for many plot types. They bring data to life, showing complex patterns and relationships clearly.
Basic Plot Types
There are key plot types for understanding data:
- Line Plots: Track trends over time
- Scatter Plots: Reveal relationships between variables
- Bar Charts: Compare categorical data
- Histograms: Show data distribution
Customizing Visualizations
Customizing plots is important. You can change:
- Color palettes
- Font styles
- Axis labels
- Grid configurations
Advanced Plotting Techniques
For deep learning and statistical modeling, advanced techniques are key. Seaborn has plots like:
- Heatmaps for correlation analysis
- Violin plots for distribution comparison
- Pair plots for multivariate relationships
Plot Type | Primary Use | Key Benefit |
---|---|---|
Scatter Plot | Relationship Detection | Visualize Variable Correlation |
Histogram | Distribution Analysis | Understand Data Spread |
Heatmap | Correlation Mapping | Identify Complex Patterns |
Learning these techniques helps turn data into insights. These insights lead to better decision-making in data science.
Advanced Data Operations and Analysis
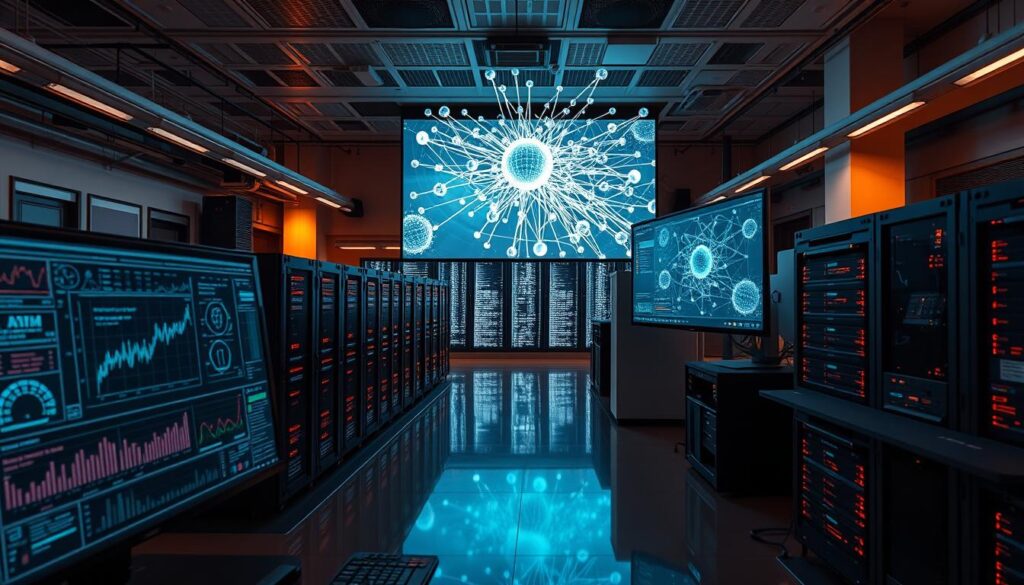
In data science, advanced operations are the top level of analysis. They turn raw data into insights that help make big decisions. Tools like Pandas and NumPy help you work with complex data, finding important information.
These operations are key for modern machine learning and AI. They let you do detailed data workups. This gets your data ready for deep analysis. Some important methods include:
- Dataset merging and joining
- Complex data reshaping
- Advanced aggregation methods
- Function application across datasets
More companies are using advanced analytics to stay ahead. A study found 53% of leaders are boosting their analytics spending. This helps streamline operations and boost productivity. For example, Canadian Tire saw a 20% sales increase using analytics during tough times.
Learning these advanced techniques can greatly improve your data science work. Predictive modeling combines machine learning and data mining. It helps predict future outcomes with high accuracy. This way, you can make better decisions that grow your business.
Advanced analytics transforms raw data into strategic insights, enabling businesses to anticipate market trends and optimize operations.
Advanced data operations are essential for anyone in business analytics, scientific research, or machine learning. They help you explore and understand data deeply.
Real-World Applications in Data Science
Data science has changed how companies tackle big challenges in many fields. It uses predictive analytics and big data to find key insights. These insights help drive new ideas and smart decisions.
Today, data science is key to solving real problems in many areas. It goes from business to scientific research.
Business Analytics Strategies
In business, data mining helps companies understand the market better. They use Python analytics for:
- Accurate sales forecasts
- Deep customer insights
- Market analysis
For example, Amazon and Netflix use data to make your experience better. They analyze what you like to watch or buy.
Scientific Research Applications
Science also relies on data science for tough research. Scientists use advanced methods to:
- Study genes
- Understand climate changes
- Make environmental predictions
Machine Learning Integration
Data science connects raw data to smart systems. It combines Pandas and NumPy with Scikit-learn. This lets researchers:
- Get data ready
- Create detailed features
- Build strong models
Data science is changing tech, from health to self-driving cars. It keeps pushing what’s possible.
Conclusion
Learning Pandas and NumPy opens up a lot in data science. These Python libraries give you key tools for handling data, doing stats, and complex calculations. They make working with neural networks and natural language processing easier.
The field of data science is always changing. The Bureau of Labor Statistics says data scientist jobs will grow by 36% from 2023 to 2033. Knowing Pandas and NumPy puts you ahead in this fast-paced area. You can work in healthcare, finance, or research, using these libraries as your base.
Data science blends stats, computer science, and specific knowledge. Being good with Pandas and NumPy helps you solve tough data problems. You can turn simple data into deep insights in many fields.
Your learning journey is just starting. Keep practicing, learning new things, and keeping up with new tech. The world of data science is vast and always changing. It offers great chances for those who are eager to learn and dive deep.