How to Build a Simple Calculator Using JavaScript
Web developers can boost their skills by making a simple calculator with JavaScript. This project is great for learning web development basics. It also helps you create a useful tool for basic math.
To make a calculator app, you need HTML for structure, CSS for looks, and JavaScript for the math. Your app will have 16 buttons for numbers, math signs, clear, and equals.
Building a simple calculator teaches you about event handling, design, and math operations. You’ll make an interface that changes as users type in their math problems.
This guide will show you how to make a small calculator. It will be 300 pixels wide, have a grid layout, and work smoothly. By following these steps, you’ll turn your knowledge into a working web app.
Get ready for a fun web development adventure. You’ll use your skills to solve real problems. Your simple calculator project is about to begin!
Table of Contents
Getting Started with Calculator Development
Starting your simple calculator project needs careful planning and the right tools. This guide will help you set up your development environment. You’ll be ready for an exciting programming journey.
Before you start coding, pick the right tools and know what your project needs. The development process has key steps. These steps will help build a strong user interface.
Setting Up Your Development Environment
Choose a professional code editor that fits your programming language. The best choices are:
- Visual Studio Code
- Sublime Text
- PyCharm
Understanding Basic Requirements
Your simple calculator project needs a clear plan. Here are the basics:
- Pick a programming language (Python 3.x is good)
- Do 4 basic arithmetic operations
- Make a clean user interface
- Add error handling
Project Structure Overview
A simple calculator project has three main files:
- index.html: It’s the structural base
- style.css: It handles the look and layout
- app.js: It makes the calculator work
By following these steps, you’ll make a professional simple calculator. It will have a user-friendly interface and meet today’s programming standards.
Creating the HTML Structure
Building a strong calculator app begins with a solid HTML base. Your user interface is where users interact most. So, its structure is key for a smooth experience.
When designing your calculator app’s HTML, focus on a detailed layout. This layout should support many functions. It will have three main parts:
- Display screen for showing calculations
- Numeric buttons (0-9)
- Operational buttons for arithmetic functions
Building the Calculator Interface
The interface starts with a container div for all calculator parts. Inside, you’ll make a display area and a button grid. This layout keeps your app clean and organized.
Adding Button Elements
Your buttons will include a wide range of interactive options. The calculator will have:
- 12 numeric buttons (0-9 and 00)
- 5 arithmetic operator buttons
- 3 special operation buttons
Implementing the Display Screen
The display screen is vital for your interface. It should show inputs and results clearly. Use an input field with the right attributes for smooth interaction and easy reading.
Pro tip: Use semantic HTML elements to create a structured and accessible calculator interface.
By building your HTML structure with care, you lay a solid base for your calculator app. This base supports smooth functionality and a great user experience.
Styling Your Simple Calculator
To make your simple calculator look good, you need to use CSS styling wisely. You want to turn a basic HTML into a calculator app that’s both pretty and easy to use.
- Container Styling
- Set container padding to 20 pixels
- Apply border-radius of 10 pixels for rounded corners
- Add subtle box-shadow with rgba(0, 0, 0, 0.1)
- Display Screen Design
- Width: 160 pixels
- Height: 40 pixels
- Clean, readable font
- Button Layout
- Grid configuration: 4 columns
- Individual button size: 40×40 pixels
- Hover effect: Background color transition
Interactive elements make the user interface more fun. Buttons change color from #4CAF50 to #45a049 when you hover over them. The equals button is special, spanning two columns with a blue background (#2196F3) that turns to #0b7dda when you click it.
Element | CSS Property | Value |
---|---|---|
Container | Vertical Centering | 100vh viewport height |
Buttons | Hover Effect | Background color transition |
Equals Button | Grid Span | 2 Columns |
By following these design tips, you’ll make a simple calculator that looks professional and is easy to use. It will be both functional and good-looking.
Essential JavaScript Functions for Calculator Operations
To make a calculator work, you need to know some key JavaScript functions. These functions are what make your calculator do math. With the right programming, your calculator will become a powerful tool.
Creating strong math functions is important. They make sure your calculator works well and does math right.
Handling Number Input
Getting numbers from users is the first step. You’ll need to write functions that:
- Catch the numbers users click
- Save these numbers
- Check if the numbers are okay
- Stop bad numbers from getting in
Managing Arithmetic Operations
JavaScript has different ways to do math. You can use if/else statements or switch cases:
Operation | Method | Example |
---|---|---|
Addition | If/Else | 5 + 3 = 8 |
Division | Switch Case | 4 ÷ 2 = 2 |
Multiplication | Conditional Check | 4 × 5 = 20 |
Subtraction | Error Handling | 10 – 6 = 4 |
Implementing Clear Functionality
The clear function lets users start over. It should:
- Clear the current math
- Wipe the screen
- Go back to the start
- Stop old data from messing things up
Learning these JavaScript functions helps you make a calculator that does math well. It will be easy for users to use.
Building the User Interface Components
Creating a good user interface for your calculator app is all about planning and detail. The interface is where users interact with your tool. You want it to be easy to use and look good.
Important parts of the calculator interface are:
- Number buttons (0-9)
- Arithmetic operator buttons (+, -, *, /)
- Special function buttons (clear, equals)
- Display screen for showing calculations
When designing, keep these tips in mind:
- Keep the button layout clean and organized.
- Use buttons that are 375 pixels wide and 667 pixels tall.
- Make the display frame responsive, at 221 pixels tall.
- Use a grid system for placing buttons.
The display should show up to 11 characters. This makes it easy to read and prevents too much information. Adding special features like square and square root buttons can make the app more useful.
Make sure buttons are easy to tell apart. They should have clear labels and be placed where they make sense. The digit buttons should follow a grid, with 0 in a spot that’s easy to reach.
By focusing on user experience, you’ll make a calculator app that’s both useful and fun to use.
Implementing Mathematical Operations
Creating a solid calculator means getting the basic math right. Your JavaScript calculator will handle four main math tasks: adding, subtracting, multiplying, and dividing. These are the building blocks of any calculator.
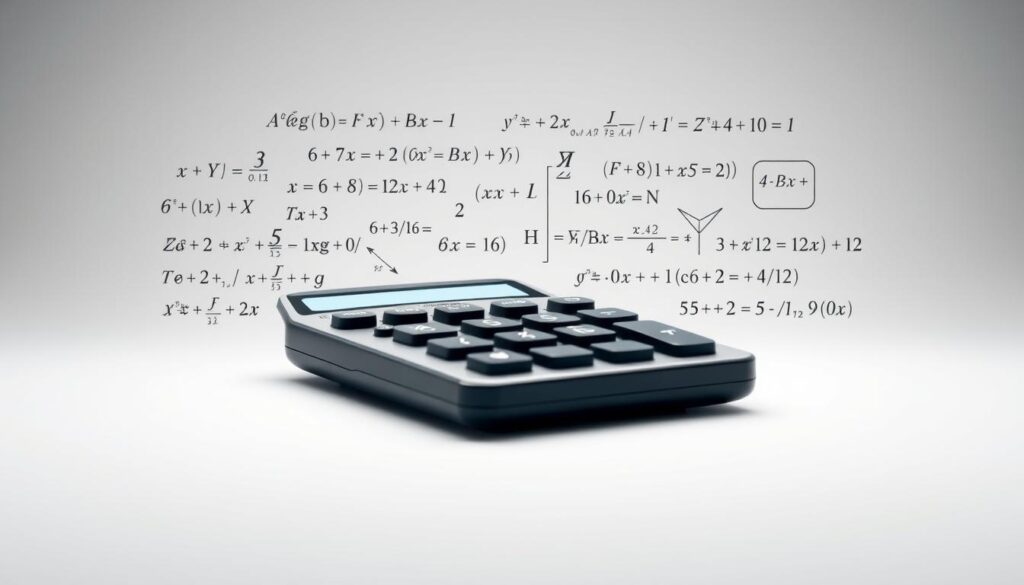
Each math task needs its own logic to get the right answers. Let’s dive into how to set these up.
Addition and Subtraction Fundamentals
Addition and subtraction are easy to do in your calculator. They involve basic number work:
- Create a function that takes two numbers
- Do the math directly
- Give back the result
For instance, an addition function could be:
function add(a, b) { return a + b; }
Multiplication and Division Techniques
Multiplication and division are a bit trickier. They need extra care to avoid mistakes:
- Watch out for dividing by zero
- Make sure inputs are numbers
- Keep an eye on decimal precision
Error Handling Strategies
Good calculators also handle errors well. They use a few key strategies:
- Catch division by zero
- Check if inputs are numbers
- Give clear error messages
By carefully setting up these math operations, you’ll make a calculator that works well. It will handle addition, subtraction, multiplication, and division accurately.
Display and Input Handling
Creating a good user interface is key for a simple calculator. The display screen is where the user and the app talk. Good design makes input and output easy and clear.
The display area needs careful planning. It’s usually 160 pixels wide and 40 pixels tall. This size lets users see inputs and results well.
- Set a fixed display width of 160 pixels
- Maintain a display height of 40 pixels
- Use clear, readable fonts
- Implement real-time input updating
Your interface needs some important parts for input handling:
- Input field for numeric entry
- Operator selection mechanism
- Display area for showing calculations
- Clear and reset functionality
Feature | Specification |
---|---|
Input Field Type | Numeric input only |
Font Size | 16 pixels |
Padding | 10 pixels |
When making the display for your calculator, aim for a user-friendly interface. Make sure each button press shows the change right away. This gives users quick feedback.
Don’t forget about error handling. Add clear messages for things like division by zero. This helps users know when something can’t be done.
Adding Interactive Features
To make a calculator app engaging, you need more than basic functions. The user interface is key to a smooth experience. Advanced interaction techniques can turn a simple calculator into a user-friendly tool.
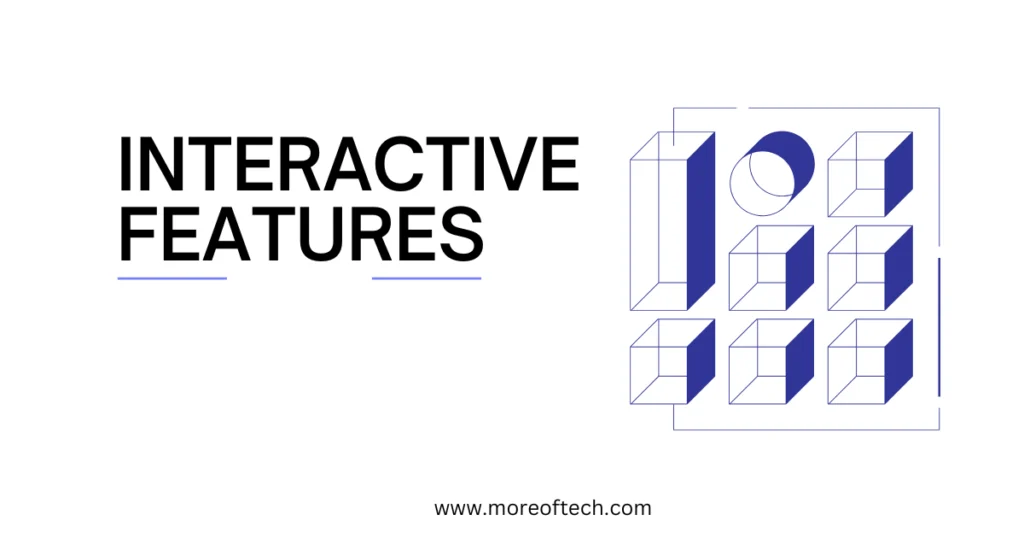
Button Click Events
Button click events are vital for a calculator app’s interactivity. JavaScript event listeners are a great way to handle user actions. Make sure to attach click events to each button for quick and smooth responses.
- Add event listeners to all numeric and operator buttons
- Implement click handlers for each button type
- Capture and process user input dynamically
Keyboard Support Implementation
Adding keyboard support makes your app more accessible. By using keyboard event listeners, you allow users to calculate with their keyboard. This makes your app more inclusive.
Key | Function |
---|---|
Number Keys (0-9) | Input numeric values |
Operator Keys (+, -, *, /) | Perform mathematical operations |
Enter/Return | Execute calculation |
Backspace | Delete last input |
Visual Feedback Elements
Visual feedback makes your calculator app interactive. Use animations, color changes, and hover effects for instant feedback. These cues show users what’s happening with their actions and the app’s state.
- Create hover states for buttons
- Add click animations
- Implement error state indicators
- Use color transitions for visual engagement
Testing and Debugging the Calculator
Creating a reliable simple calculator needs thorough testing and strategic debugging. Your journey to making a strong app involves checking every part of your basic calculations.
Begin by adding good error handling strategies. Look at key scenarios that could hurt your calculator’s performance:
- Division by zero prevention
- Input validation checks
- Handling unexpected user interactions
- Managing complex mathematical scenarios
Debugging your simple calculator needs a careful plan. Use browser developer tools to check JavaScript code and find issues fast. Set breakpoints to follow the execution flow and see how operations work together.
Testing Area | Key Focus | Potential Issues |
---|---|---|
Number Input | Validate numeric entries | Non-numeric inputs |
Arithmetic Operations | Check calculation accuracy | Precision errors |
Error Handling | Manage edge cases | Division by zero |
Focus on edge cases that could mess up your basic calculations. Use defensive programming to keep your simple calculator stable under different inputs.
Remember: Rigorous testing turns a basic calculator into a reliable tool.
Think about making a detailed test suite for many scenarios. This will help you find and fix bugs before you release your calculator app.
Enhancing Calculator Performance
To make your calculator better, focus on improving arithmetic functions and math operations. Use advanced techniques to speed up calculations. This will make your calculator work faster and feel more responsive.
Think about adding more features to your calculator. It could do more than just simple math. Add memory, percentage tools, and support for scientific functions. This will make your calculator more useful and interesting for users.
Improving your calculator’s performance means making your code better. Look for slow spots in your math operations. Add error handling to give users helpful feedback and avoid crashes. By doing this, you’ll make a calculator that’s strong and meets many needs.
Use the latest JavaScript methods to make your calculator faster. Use browser tools to check how fast your code runs. Look for ways to speed up repetitive tasks and manage memory well. Your aim is to have a calculator that’s quick, accurate, and uses less resources.